Installation
Using Google App Engine requires having the Google Cloud SDK installed on your local machine. This SDK allows for deployment of the application into Google App Engine.
Windows installation: https://cloud.google.com/sdk/docs/quickstart-windows
Linux (Ubuntu) installation: https://cloud.google.com/sdk/docs/downloads-apt-get
Mac installation: https://cloud.google.com/sdk/docs/quickstart-macos
Additional steps before being able to deploy an app include:
- Setting up a billing account
- Authentication
- Creation of a project
Deploying an app
Supplementary materials
Flask
Flask Homepage: https://palletsprojects.com/p/flask/
Getting started with flask:
- https://blog.miguelgrinberg.com/post/the-flask-mega-tutorial-part-i-hello-world
- https://realpython.com/tutorials/flask/
- https://www.fullstackpython.com/flask.html
App Engine: Standard vs Flexible
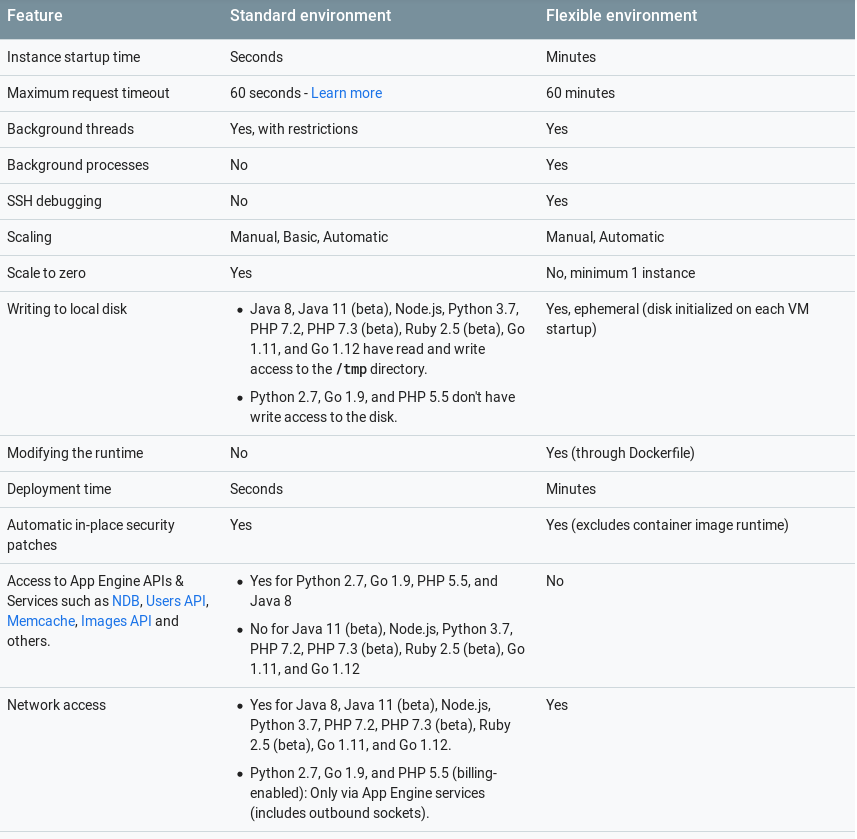
Python app engine (flexible) examples
Github repository: https://github.com/GoogleCloudPlatform/python-docs-samples/tree/master/appengine/flexible
Deploying an app to App Engine
The most basic app you can deploy on app engine requires 3 files:
- app.yaml
- main.py
- requirements.txt
If you are planning on running functions on a schedule you will need to include a 4th file:
- cron.yaml
app.yaml
app.yaml reference: https://cloud.google.com/appengine/docs/flexible/python/reference/app-yaml
runtime: python
env: flex
entrypoint: gunicorn -b :$PORT main:app
runtime_config:
python_version: 3
manual_scaling:
instances: 1
resources:
cpu: 1
memory_gb: 0.5
disk_size_gb: 10
main.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return 'Hello World!'
@app.route('/bye')
def goodbye():
return 'Goodbye World!'
requirements.txt
requirements.txt reference: https://pip.readthedocs.io/en/1.1/requirements.html
Flask==1.0.2
gunicorn==19.9.0
cron.yaml
cron.yaml reference: https://cloud.google.com/appengine/docs/standard/python/config/cronref
cron:
- description: "daily summary job"
url: /tasks/summary
schedule: every 24 hours
- description: "monday goodbye"
url: /bye
schedule: every monday 09:00
timezone: Australia/NSW
Useful Google Cloud SDK commands
To set up configuration once first installed
gcloud init
Change the active project that will be interacted with
gcloud config set project PROJECT_ID
Deploying app to app engine
gcloud app deploy
Extending basic functionality
Utilising other Google cloud services
- Google Cloud Storage: https://github.com/GoogleCloudPlatform/python-docs-samples/tree/master/appengine/flexible/storage
- Google Cloud SQL: https://github.com/GoogleCloudPlatform/python-docs-samples/tree/master/appengine/flexible/cloudsql
Interacting with slack:
And some helper code to get up and running interacting with slack
from dotenv import load_dotenv
import os
import slack
import requests
# load files in .env file as environment variables.
load_dotenv()
def post_to_random_channel(some_text):
'''
Posts 'some_text' into the #random channel
'''
# Authenticate client
client = slack.WebClient(token = os.environ['token'])
# post text
response = client.chat_postMessage(
channel = '#random',
text = some_text
)
assert response["ok"]
return None
def get_bitcoin_price(country_code = 'USD'):
'''
Query coindesk API to get current bitcoin price.
'''
# collect response
r = requests.get("https://api.coindesk.com/v1/bpi/currentprice.json")
# extract price from response
price = r.json()['bpi'][country_code]['rate']
return price
def post_bitcoin_price():
price = get_bitcoin_price()
text = 'The price of bitcoin is currently USD${}'.format(price)
post_to_random_channel(text)
return None
def upload_to_random(file_path = 'stream.jpg'):
'''
uploads file at file_path to a the
#random slack channel
'''
client = slack.WebClient(token = os.environ['token'])
response = client.files_upload(
channels='#random',
file=file_path)
assert response["ok"]
return None
Interacting with Twitter: