A lightweight example of saving some logs to an SQLite database in a python script.
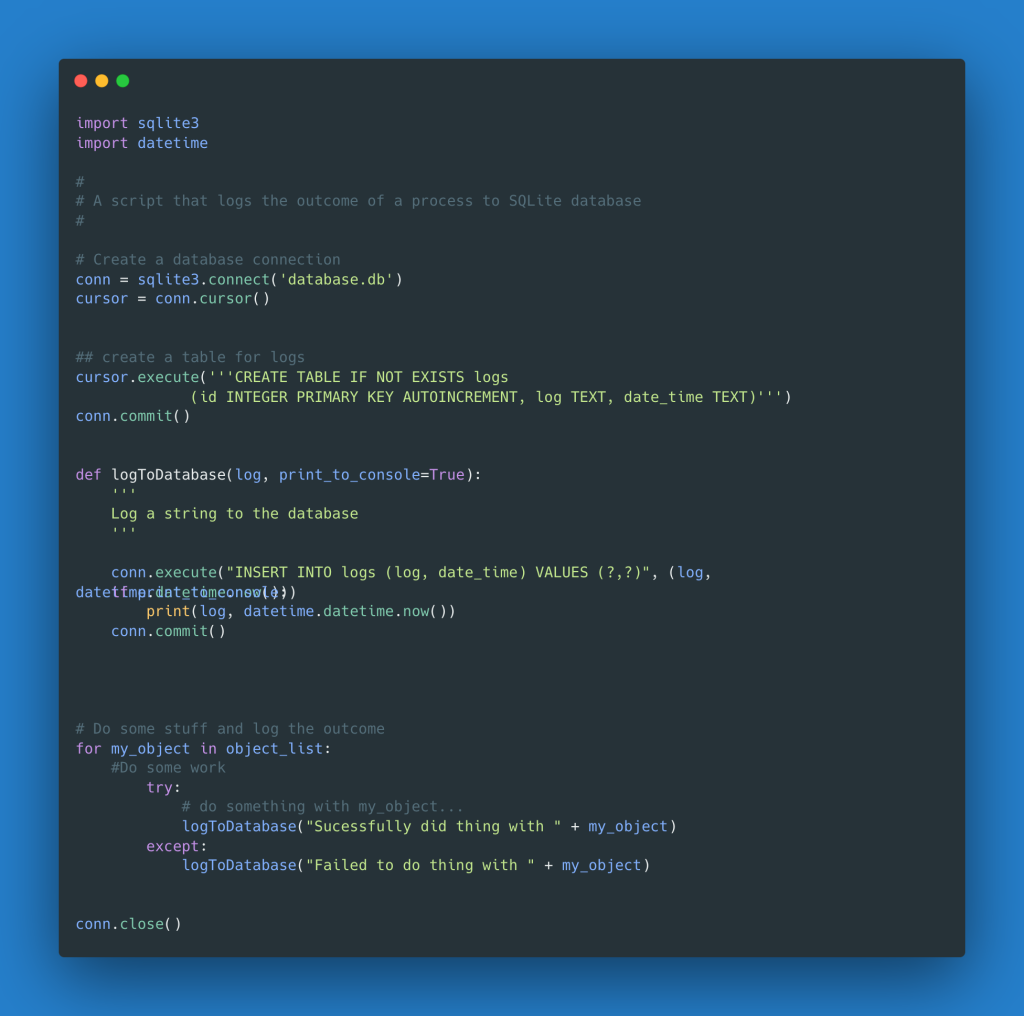
import sqlite3
import datetime
#
# A script that logs the outcome of a process to SQLite database
#
# Create a database connection
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
## create a table for logs
cursor.execute('''CREATE TABLE IF NOT EXISTS logs
(id INTEGER PRIMARY KEY AUTOINCREMENT, log TEXT, date_time TEXT)''')
conn.commit()
def logToDatabase(log, print_to_console=True):
'''
Log a string to the database
'''
conn.execute("INSERT INTO logs (log, date_time) VALUES (?,?)", (log, datetime.datetime.now()))
if print_to_console:
print(log, datetime.datetime.now())
conn.commit()
# Do some stuff and log the outcome
for my_object in object_list:
#Do some work
try:
# do something with my_object...
logToDatabase("Sucessfully did thing with " + my_object)
except:
logToDatabase("Failed to do thing with " + my_object)
conn.close()