A short guide to adding user authentication to Streamlit apps.
So you’ve created a beautiful Streamlit app which your users love, but only certain people should be able to access and use this new tool. This is where adding authentication to limit access can help.
Inspiration:
- https://blog.streamlit.io/streamlit-authenticator-part-1-adding-an-authentication-component-to-your-app/
- https://github.com/mkhorasani/Streamlit-Authenticator?ref=blog.streamlit.io
Install libraries
pip install streamlit streamlit-authenticator
Generate a password hash for the user of your Streamlit application. Use Python to run the Hasher
function inside the streamlit-authenticator library.
import streamlit_authenticator
#
password = 'the_password_to_login'
stauth.authenticate.authentication.Hasher([password]).generate()
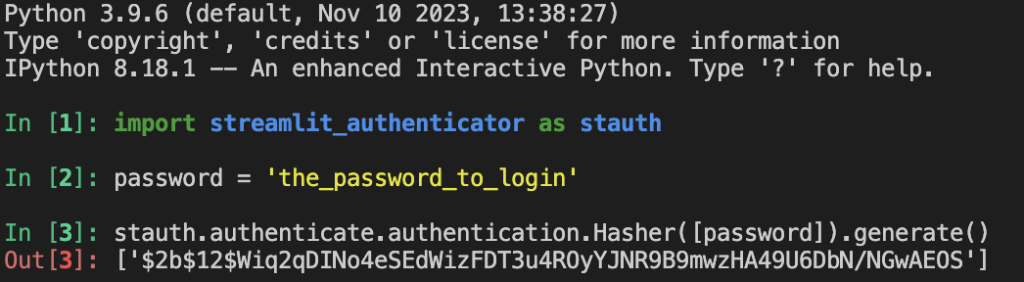
Take the hashed password and insert it the config YAML file that is used to manage users to the app.
config.yaml
:
credentials:
usernames:
user:
email: msmith@gmail.com
name: marquin
password: $2b$12$Wiq2qDINo4eSEdWizFDT3u4ROyYJNR9B9mwzHA49U6DbN/NGwAEOS
paul:
email: paul@gmail.com
name: Paul Smith
password: $2b$12$upzqGiuKBniDRjCmazaB4.Xbid5CUO2EfNlJe8oJ.Nl/4E6irZmjS
cookie:
expiry_days: 30
key: random_signature_key # Must be string
name: random_cookie_name
This config.yaml
file contains the information for 2 users. The username is ‘user’ for the first, and the username for the second is ‘paul’. More users will need to be added to this config YAML file for more users to be able to log in to the Streamlit application.
The password for ‘paul’ is the result of hashing ‘abc’ with the Hasher
function from the streamlit-authenticator python library.
The Streamlit app python file would look like this to implement user authentication
app.py
import streamlit as st
import streamlit_authenticator as stauth
import yaml
from yaml.loader import SafeLoader
with open('config.yaml') as file:
config = yaml.load(file, Loader=SafeLoader)
authenticator = stauth.Authenticate(
config['credentials'],
config['cookie']['name'],
config['cookie']['key'],
config['cookie']['expiry_days']
)
authenticator.login()
if st.session_state["authentication_status"]:
authenticator.logout()
st.write(f'Welcome *{st.session_state["name"]}*')
st.title('Some content')
# enter the rest of the streamlit app here
elif st.session_state["authentication_status"] is False:
st.error('Username/password is incorrect')
elif st.session_state["authentication_status"] is None:
st.warning('Please enter your username and password')
Running the Streamlit application streamlit run app.py
will now show a login form:
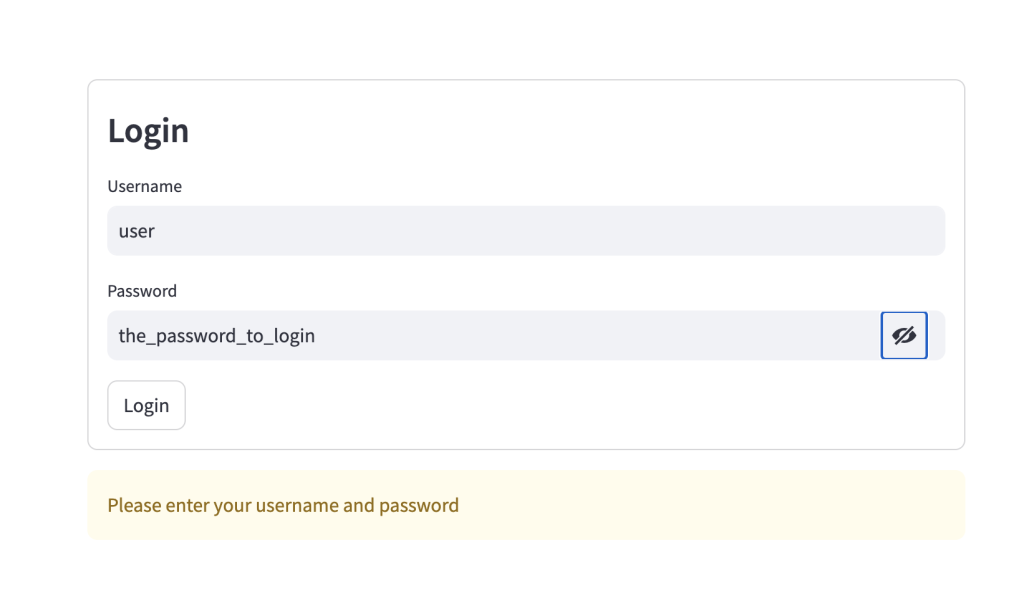
A successful login for this toy example looks like this:
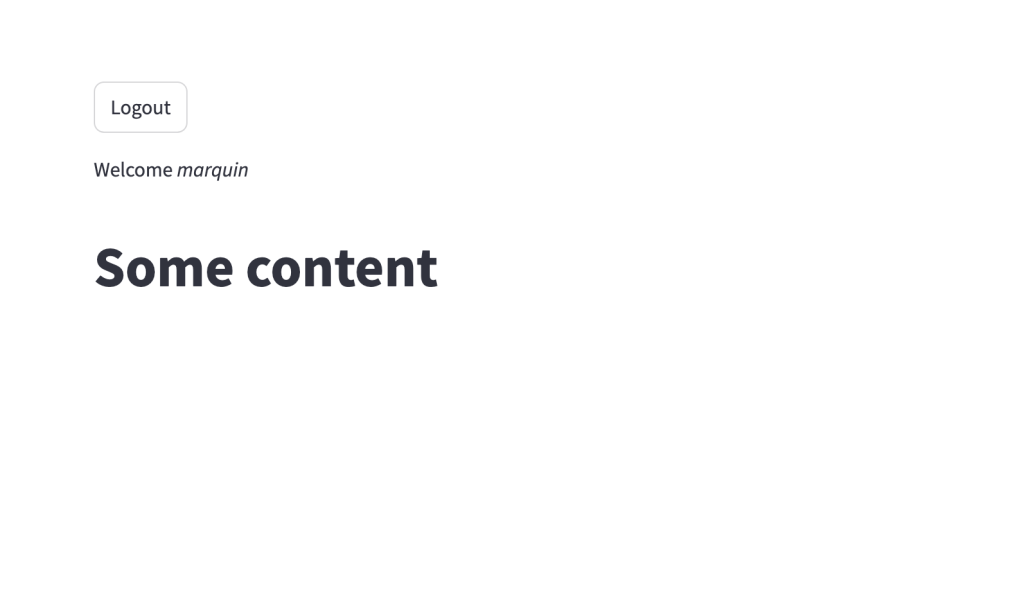